Easy Process Management with PM2
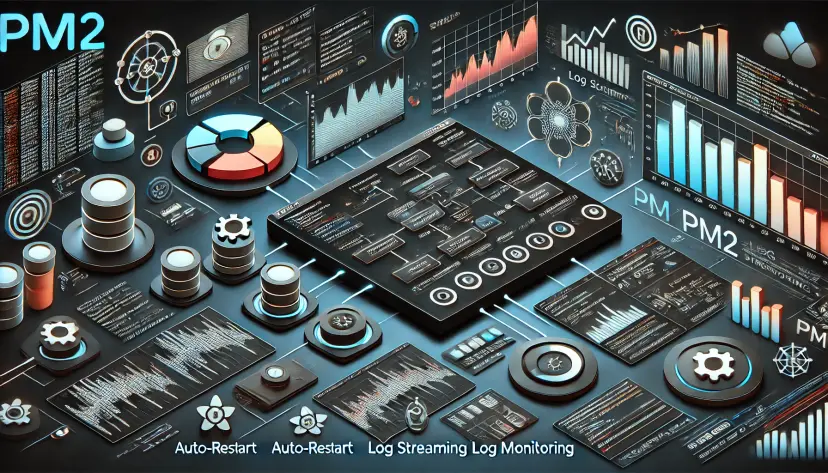
PM2 is a program that makes managing processes simple. It allows log streaming from multiple processes, auto-restart when a process exits, scheduling, visualization tools and more. We could manually manage our processes by running manual health checks and restarting processes when they don’t respond, but adding this logic to your codebase can be extraneous. Let a process manager do the work for you!
Setup
First, let’s install PM2. We can do this multiple ways, but if you have node, you can run npm i -g pm2
. When we use the -g
flag with npm it installs the library globally so that we can use it without installing it in a specific project. Sweet.
Starting a Process
By default, PM2 has process restart logic. If we don’t pass a flag, once a process exits, PM2 will restart it. For one-off scripts, this is not the functionality we want. To only run a process once, we can use the --no-autorestart
flag.
pm2 start --no-autorestart --name "$source->$destination" node -- src/scraper/scraper.js --from "SFO" --to "JFK" --date "2023-11-07"
Viewing Processes
We can view processes and details about them through pm2 list
, which lists processes with details at the current time.
If you want to monitor processes continually, pm2 monit
would be a better fit. The process monitor has split panes that shows up to date details on each process and a pane which allows you to inspect output from the process.
Stopping Processes
If we have long running processes that we want to stop, we can run pm2 stop <process-name>
.
Kill all Processes
If we have a ton of stale processes, we can remove them from our process list by running pm2 kill
Process Management + Bash Scripts
Now that we have the ability to easily kick off processes and manage them, we can use a bash script to loop over airports and kick off jobs.
#!/bin/bash
declare -a airports=(
"AMS"
"ATL"
"SFO"
"IST"
)
for source in "${airports[@]}"; do
for destination in "${airports[@]}"; do
if [[ "$source" == "$destination" ]]; then
continue
fi
echo "Searcing $source->$destination"
pm2 start --no-autorestart --name "$source->$destination" node -- src/scraper/scraper.js --from "$source" --to "$destination" --date "2023-11-07"
done
done